datum 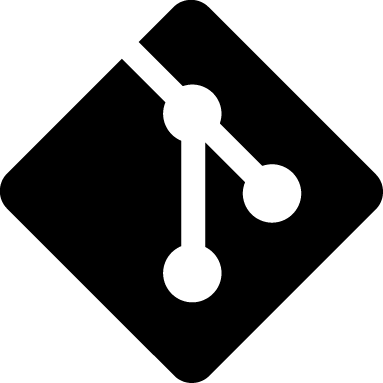
The absolute base class for everything
A datum instantiated has no physical world presence, use an atom if you want something that actually lives in the world
Be very mindful about adding variables to this class, they are inherited by every single thing in the entire game, and so you can easily cause memory usage to rise a lot with careless use of variables at this level
Vars | |
_active_timers | Active timers with this datum as the target |
---|---|
_datum_components | Components attached to this datum |
_listen_lookup | Any datum registered to receive signals from this datum is in this list |
_signal_procs | Lazy associated list in the structure of target -> list(signal -> proctype) that are run when the datum receives that signal |
_status_traits | Status traits attached to this datum. associative list of the form: list(trait name (string) = list(source1, source2, source3,...)) |
datum_flags | Datum level flags |
filter_data | List for handling persistent filters. |
gc_destroyed | Tick count time when this object was destroyed. |
layout_prefs_used | The layout pref we take from the player looking at this datum's UI to know what layout to give. |
open_uis | Open uis owned by this datum Lazy, since this case is semi rare |
tgui_shared_states | global |
weak_reference | A weak reference to another datum |
Procs | |
AddElementTrait | Used to manage (typically non_bespoke) elements with multiple sources through traits so we don't have to make them a components again. The element will be later removed once all trait sources are gone, there's no need of a "RemoveElementTrait" counterpart. |
Destroy | Default implementation of clean-up code. |
GenerateTag | Generate a tag for this /datum, if it implements one Should be called as early as possible, best would be in New, to avoid weakref mistargets Really just don't use this, you don't need it, global lists will do just fine MOST of the time We really only use it for mobs to make id'ing people easier |
GetComponent | Return any component assigned to this datum of the given type |
GetComponents | Get all components of a given type that are attached to this datum |
GetExactComponent | Return any component assigned to this datum of the exact given type |
REPLACE_PRONOUNS | A proc to replace pronouns in a string with the appropriate pronouns for a target atom. Uses associative list access from a __DEFINE list, since associative access is slightly faster |
RegisterSignal | Register to listen for a signal from the passed in target |
RegisterSignals | Registers multiple signals to the same proc. |
RemoveComponentSource | Removes a component source from this datum |
TakeComponent | Transfer this component to another parent |
Topic | Called when a href for this datum is clicked |
TransferComponents | Transfer all components to target |
UnregisterSignal | Stop listening to a given signal from target |
_AddComponent | Creates an instance of new_type in the datum and attaches to it as parent |
_AddElement | Finds the singleton for the element type given and attaches it to src |
_LoadComponent | Get existing component of type, or create it and return a reference to it |
_RemoveElement | Finds the singleton for the element type given and detaches it from src You only need additional arguments beyond the type if you're using ELEMENT_BESPOKE |
_SendSignal | Internal proc to handle most all of the signaling procedure |
_clear_signal_refs | Only override this if you know what you're doing. You do not know what you're doing This is a threat |
add_filter | |
add_filters | A version of add_filter that takes a list of filters to add rather than being individual, to limit calls to update_filters(). |
add_traits | Proc that handles adding multiple traits to a target via a list. Must have a common source and target. |
change_filter_priority | Updates the priority of the passed filter key |
deserialize_json | Deserializes from JSON. Does not parse type. |
deserialize_list | Accepts a LIST from deserialize_datum. Should return whether or not the deserialization was successful. |
dump_harddel_info | Return text from this proc to provide extra context to hard deletes that happen to it Optional, you should use this for cases where replication is difficult and extra context is required Can be called more then once per object, use harddel_deets_dumped to avoid duplicate calls (I am so sorry) |
get_filter | Returns the filter associated with the passed key |
get_filter_index | Returns the indice in filters of the given filter name. If it is not found, returns null. |
jatum_new_arglist | Gets the flat list that can be passed in a new /type(argslist(retval)) expression to recreate the datum. Must only return a list containing values that can be JATUM serialized |
modify_filter | |
process | This proc is called on a datum on every "cycle" if it is being processed by a subsystem. The time between each cycle is determined by the subsystem's "wait" setting. You can start and stop processing a datum using the START_PROCESSING and STOP_PROCESSING defines. |
ref_search_details | Return info about us for reference searching purposes Will be logged as a representation of this datum if it's a part of a search chain |
remove_filter | Removes the passed filter, or multiple filters, if supplied with a list. |
remove_traits | Proc that handles removing multiple traits from a target via a list. Must have a common source and target. |
selfdelete | Calls qdel on itself, because signals dont allow callbacks |
serialize_json | Serializes into JSON. Does not encode type. |
serialize_list | Return a list of data which can be used to investigate the datum, also ensure that you set the semver in the options list |
string_assoc_list | Caches associative lists with non-numeric stringify-able index keys and stringify-able values (text/typepath -> text/path/number). |
string_assoc_nested_list | Caches associative nested lists with non-numeric stringify-able index keys and stringify-able values (text/typepath -> text/path/number). |
string_numbers_list | Caches lists of numeric values. |
transition_filter | |
transition_filter_chain | |
ui_act | public |
ui_assets | public |
ui_close | public |
ui_data | public |
ui_host | private |
ui_interact | public |
ui_state | private |
ui_static_data | public |
ui_status | public |
update_filters | Reapplies all the filters. |
update_static_data | public |
update_static_data_for_all_viewers | public |
vv_do_topic | This proc is only called if everything topic-wise is verified. The only verifications that should happen here is things like permission checks! href_list is a reference, modifying it in these procs WILL change the rest of the proc in topic.dm of admin/view_variables! This proc is for "high level" actions like admin heal/set species/etc/etc. The low level debugging things should go in admin/view_variables/topic_basic.dm in case this runtimes. |
vv_edit_var | Called when a var is edited with the new value to change to |
vv_get_dropdown | Gets all the dropdown options in the vv menu. When overriding, make sure to call . = ..() first and append to the result, that way parent items are always at the top and child items are further down. Add separators by doing VV_DROPDOWN_OPTION("", "---") |
Var Details
_active_timers 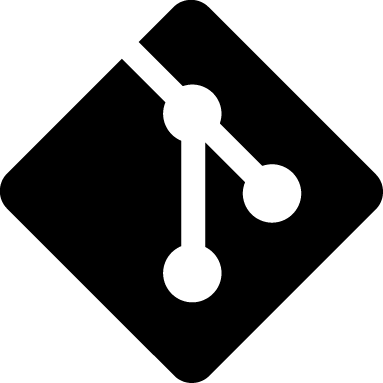
Active timers with this datum as the target
_datum_components 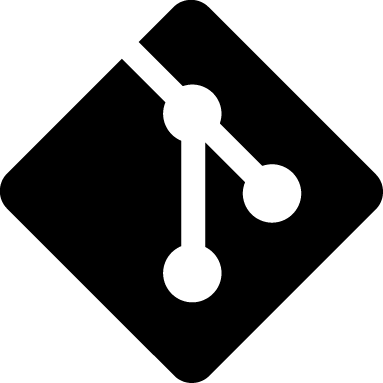
Components attached to this datum
Lazy associated list in the structure of type -> component/list of components
_listen_lookup 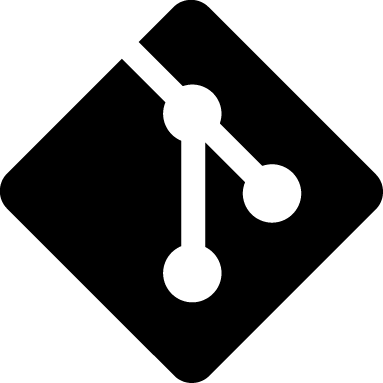
Any datum registered to receive signals from this datum is in this list
Lazy associated list in the structure of signal -> registree/list of registrees
_signal_procs 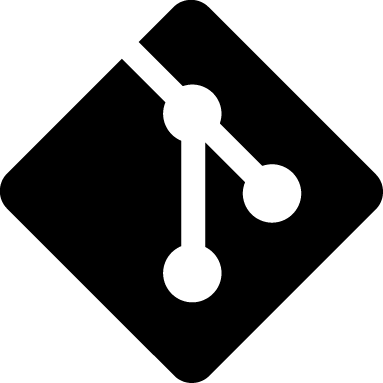
Lazy associated list in the structure of target -> list(signal -> proctype)
that are run when the datum receives that signal
_status_traits 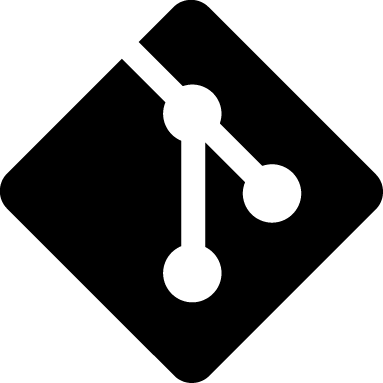
Status traits attached to this datum. associative list of the form: list(trait name (string) = list(source1, source2, source3,...))
datum_flags 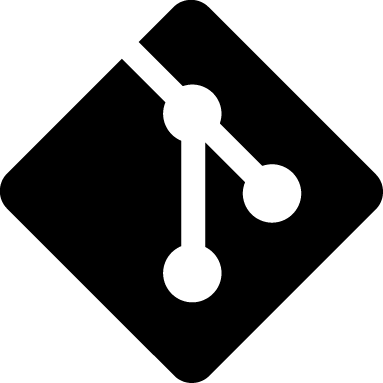
Datum level flags
filter_data 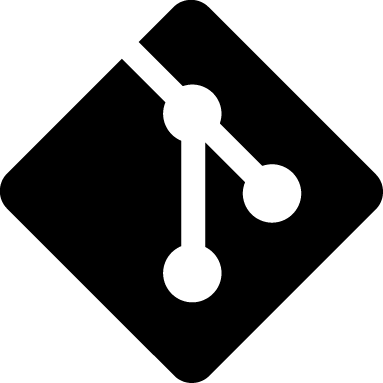
List for handling persistent filters.
gc_destroyed 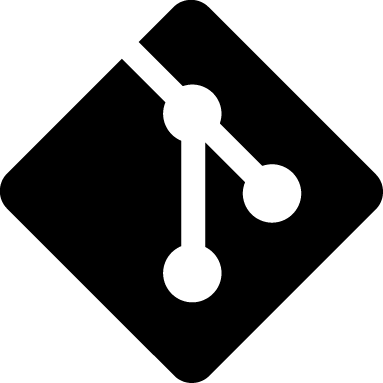
Tick count time when this object was destroyed.
If this is non zero then the object has been garbage collected and is awaiting either a hard del by the GC subsystme, or to be autocollected (if it has no references)
layout_prefs_used 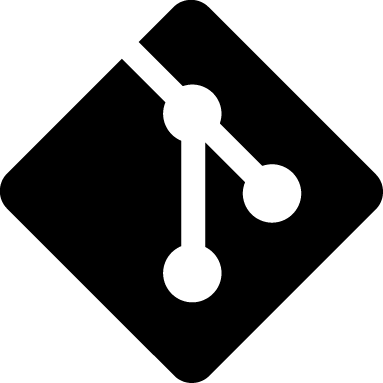
The layout pref we take from the player looking at this datum's UI to know what layout to give.
open_uis 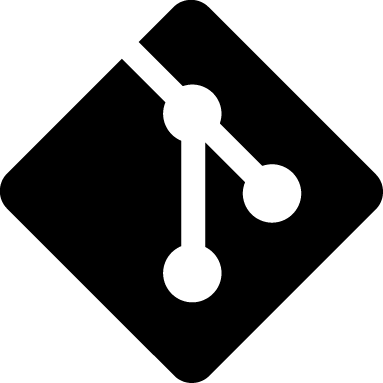
Open uis owned by this datum Lazy, since this case is semi rare
tgui_shared_states 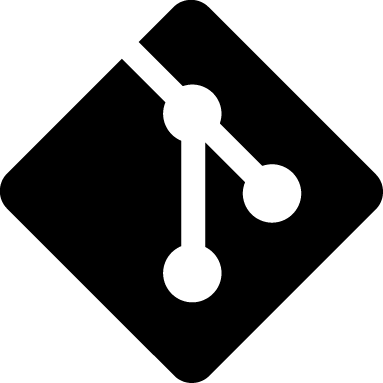
global
Associative list of JSON-encoded shared states that were set by tgui clients.
weak_reference 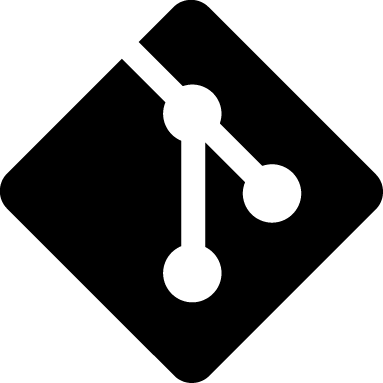
A weak reference to another datum
Proc Details
AddElementTrait
Used to manage (typically non_bespoke) elements with multiple sources through traits so we don't have to make them a components again. The element will be later removed once all trait sources are gone, there's no need of a "RemoveElementTrait" counterpart.
Destroy
Default implementation of clean-up code.
This should be overridden to remove all references pointing to the object being destroyed, if you do override it, make sure to call the parent and return its return value by default
Return an appropriate QDEL_HINT to modify handling of your deletion; in most cases this is QDEL_HINT_QUEUE.
The base case is responsible for doing the following
- Erasing timers pointing to this datum
- Erasing compenents on this datum
- Notifying datums listening to signals from this datum that we are going away
Returns QDEL_HINT_QUEUE
GenerateTag
Generate a tag for this /datum, if it implements one Should be called as early as possible, best would be in New, to avoid weakref mistargets Really just don't use this, you don't need it, global lists will do just fine MOST of the time We really only use it for mobs to make id'ing people easier
GetComponent
Return any component assigned to this datum of the given type
This will throw an error if it's possible to have more than one component of that type on the parent
Arguments:
- datum/component/c_type The typepath of the component you want to get a reference to
GetComponents
Get all components of a given type that are attached to this datum
Arguments:
- c_type The component type path
GetExactComponent
Return any component assigned to this datum of the exact given type
This will throw an error if it's possible to have more than one component of that type on the parent
Arguments:
- datum/component/c_type The typepath of the component you want to get a reference to
REPLACE_PRONOUNS
A proc to replace pronouns in a string with the appropriate pronouns for a target atom. Uses associative list access from a __DEFINE list, since associative access is slightly faster
RegisterSignal
Register to listen for a signal from the passed in target
This sets up a listening relationship such that when the target object emits a signal the source datum this proc is called upon, will receive a callback to the given proctype Use PROC_REF(procname), TYPE_PROC_REF(type,procname) or GLOBAL_PROC_REF(procname) macros to validate the passed in proc at compile time. PROC_REF for procs defined on current type or its ancestors, TYPE_PROC_REF for procs defined on unrelated type and GLOBAL_PROC_REF for global procs. Return values from procs registered must be a bitfield
Arguments:
- datum/target The target to listen for signals from
- signal_type A signal name
- proctype The proc to call back when the signal is emitted
- override If a previous registration exists you must explicitly set this
RegisterSignals
Registers multiple signals to the same proc.
RemoveComponentSource
Removes a component source from this datum
TakeComponent
Transfer this component to another parent
Component is taken from source datum
Arguments:
- datum/component/target Target datum to transfer to
Topic
Called when a href for this datum is clicked
Sends a COMSIG_TOPIC signal
TransferComponents
Transfer all components to target
All components from source datum are taken
Arguments:
- /datum/target the target to move the components to
UnregisterSignal
Stop listening to a given signal from target
Breaks the relationship between target and source datum, removing the callback when the signal fires
Doesn't care if a registration exists or not
Arguments:
- datum/target Datum to stop listening to signals from
- sig_typeor_types Signal string key or list of signal keys to stop listening to specifically
_AddComponent
Creates an instance of new_type
in the datum and attaches to it as parent
Sends the COMSIG_COMPONENT_ADDED signal to the datum
Returns the component that was created. Or the old component in a dupe situation where COMPONENT_DUPE_UNIQUE was set
If this tries to add a component to an incompatible type, the component will be deleted and the result will be null
. This is very unperformant, try not to do it
Properly handles duplicate situations based on the dupe_mode
var
_AddElement
Finds the singleton for the element type given and attaches it to src
_LoadComponent
Get existing component of type, or create it and return a reference to it
Use this if the item needs to exist at the time of this call, but may not have been created before now
Arguments:
- component_type The typepath of the component to create or return
- ... additional arguments to be passed when creating the component if it does not exist
_RemoveElement
Finds the singleton for the element type given and detaches it from src You only need additional arguments beyond the type if you're using ELEMENT_BESPOKE
_SendSignal
Internal proc to handle most all of the signaling procedure
Will runtime if used on datums with an empty lookup list
Use the SEND_SIGNAL define instead
_clear_signal_refs
Only override this if you know what you're doing. You do not know what you're doing This is a threat
add_filter
-
Add a filter to the datum.
-
This is on datum level, despite being most commonly / primarily used on atoms, so that filters can be applied to images / mutable appearances.
-
Can also be used to assert a filter's existence. I.E. update a filter regardless if it exists or not.
-
Arguments:
-
- name - Filter name
-
- priority - Priority used when sorting the filter.
-
- params - Parameters of the filter.
add_filters
A version of add_filter that takes a list of filters to add rather than being individual, to limit calls to update_filters().
add_traits
Proc that handles adding multiple traits to a target via a list. Must have a common source and target.
change_filter_priority
Updates the priority of the passed filter key
deserialize_json
Deserializes from JSON. Does not parse type.
deserialize_list
Accepts a LIST from deserialize_datum. Should return whether or not the deserialization was successful.
dump_harddel_info
Return text from this proc to provide extra context to hard deletes that happen to it Optional, you should use this for cases where replication is difficult and extra context is required Can be called more then once per object, use harddel_deets_dumped to avoid duplicate calls (I am so sorry)
get_filter
Returns the filter associated with the passed key
get_filter_index
Returns the indice in filters of the given filter name. If it is not found, returns null.
jatum_new_arglist
Gets the flat list that can be passed in a new /type(argslist(retval))
expression to recreate the datum. Must only return a list containing values that can be JATUM serialized
modify_filter
-
Update a filter's parameter to the new one. If the filter doesn't exist we won't do anything.
-
Arguments:
-
- name - Filter name
-
- new_params - New parameters of the filter
-
- overwrite - TRUE means we replace the parameter list completely. FALSE means we only replace the things on new_params.
process
This proc is called on a datum on every "cycle" if it is being processed by a subsystem. The time between each cycle is determined by the subsystem's "wait" setting. You can start and stop processing a datum using the START_PROCESSING and STOP_PROCESSING defines.
Since the wait setting of a subsystem can be changed at any time, it is important that any rate-of-change that you implement in this proc is multiplied by the seconds_per_tick that is sent as a parameter, Additionally, any "prob" you use in this proc should instead use the SPT_PROB define to make sure that the final probability per second stays the same even if the subsystem's wait is altered. Examples where this must be considered:
- Implementing a cooldown timer, use
mytimer -= seconds_per_tick
, notmytimer -= 1
. This way,mytimer
will always have the unit of seconds - Damaging a mob, do
L.adjustFireLoss(20 * seconds_per_tick)
, notL.adjustFireLoss(20)
. This way, the damage per second stays constant even if the wait of the subsystem is changed - Probability of something happening, do
if(SPT_PROB(25, seconds_per_tick))
, notif(prob(25))
. This way, if the subsystem wait is e.g. lowered, there won't be a higher chance of this event happening per second
If you override this do not call parent, as it will return PROCESS_KILL. This is done to prevent objects that dont override process() from staying in the processing list
ref_search_details
Return info about us for reference searching purposes Will be logged as a representation of this datum if it's a part of a search chain
remove_filter
Removes the passed filter, or multiple filters, if supplied with a list.
remove_traits
Proc that handles removing multiple traits from a target via a list. Must have a common source and target.
selfdelete
Calls qdel on itself, because signals dont allow callbacks
serialize_json
Serializes into JSON. Does not encode type.
serialize_list
Return a list of data which can be used to investigate the datum, also ensure that you set the semver in the options list
string_assoc_list
Caches associative lists with non-numeric stringify-able index keys and stringify-able values (text/typepath -> text/path/number).
string_assoc_nested_list
Caches associative nested lists with non-numeric stringify-able index keys and stringify-able values (text/typepath -> text/path/number).
string_numbers_list
Caches lists of numeric values.
transition_filter
-
Update a filter's parameter and animate this change. If the filter doesn't exist we won't do anything.
-
Basically a datum/proc/modify_filter call but with animations. Unmodified filter parameters are kept.
-
Arguments:
-
- name - Filter name
-
- new_params - New parameters of the filter
-
- time - time arg of the BYOND animate() proc.
-
- easing - easing arg of the BYOND animate() proc.
-
- loop - loop arg of the BYOND animate() proc.
transition_filter_chain
- Similar to transition_filter(), except it creates an animation chain that moves between a list of states.
- Arguments:
-
- name - Filter name
-
- num_loops - Amount of times the chain loops. INDEFINITE = Infinite
-
- ... - a list of each link in the animation chain. Use FilterChainStep(params, duration, easing) for each link
- Example use:
-
- add_filter("blue_pulse", 1, color_matrix_filter(COLOR_WHITE))
-
- transition_filter_chain(src, "blue_pulse", INDEFINITE,
-
- FilterChainStep(color_matrix_filter(COLOR_BLUE), 10 SECONDS, CUBIC_EASING),
-
- FilterChainStep(color_matrix_filter(COLOR_WHITE), 10 SECONDS, CUBIC_EASING))
- The above code would edit a color_matrix_filter() to slowly turn blue over 10 seconds before returning back to white 10 seconds after, repeating this chain forever.
ui_act
public
Called on a UI when the UI receieves a href. Think of this as Topic().
required action string The action/button that has been invoked by the user. required params list A list of parameters attached to the button.
return bool If the user's input has been handled and the UI should update.
ui_assets
public
Called on an object when a tgui object is being created, allowing you to push various assets to tgui, for examples spritesheets.
return list List of asset datums or file paths.
ui_close
public
Called on a UI's object when the UI is closed, not to be confused with client/verb/uiclose(), which closes the ui window
ui_data
public
Data to be sent to the UI. This must be implemented for a UI to work.
required user mob The mob interacting with the UI.
return list Data to be sent to the UI.
ui_host
private
The UI's host object (usually src_object). This allows modules/datums to have the UI attached to them, and be a part of another object.
ui_interact
public
Used to open and update UIs. If this proc is not implemented properly, the UI will not update correctly.
required user mob The mob who opened/is using the UI. optional ui datum/tgui The UI to be updated, if it exists.
ui_state
private
The UI's state controller to be used for created uis This is a proc over a var for memory reasons
ui_static_data
public
Static Data to be sent to the UI.
Static data differs from normal data in that it's large data that should be sent infrequently. This is implemented optionally for heavy uis that would be sending a lot of redundant data frequently. Gets squished into one object on the frontend side, but the static part is cached.
required user mob The mob interacting with the UI.
return list Static Data to be sent to the UI.
ui_status
public
Checks the UI state for a mob.
required user mob The mob who opened/is using the UI. required state datum/ui_state The state to check.
return UI_state The state of the UI.
update_filters
Reapplies all the filters.
update_static_data
public
Forces an update on static data. Should be done manually whenever something happens to change static data.
required user the mob currently interacting with the ui optional ui ui to be updated
update_static_data_for_all_viewers
public
Will force an update on static data for all viewers. Should be done manually whenever something happens to change static data.
vv_do_topic
This proc is only called if everything topic-wise is verified. The only verifications that should happen here is things like permission checks! href_list is a reference, modifying it in these procs WILL change the rest of the proc in topic.dm of admin/view_variables! This proc is for "high level" actions like admin heal/set species/etc/etc. The low level debugging things should go in admin/view_variables/topic_basic.dm in case this runtimes.
vv_edit_var
Called when a var is edited with the new value to change to
vv_get_dropdown
Gets all the dropdown options in the vv menu. When overriding, make sure to call . = ..() first and append to the result, that way parent items are always at the top and child items are further down. Add separators by doing VV_DROPDOWN_OPTION("", "---")